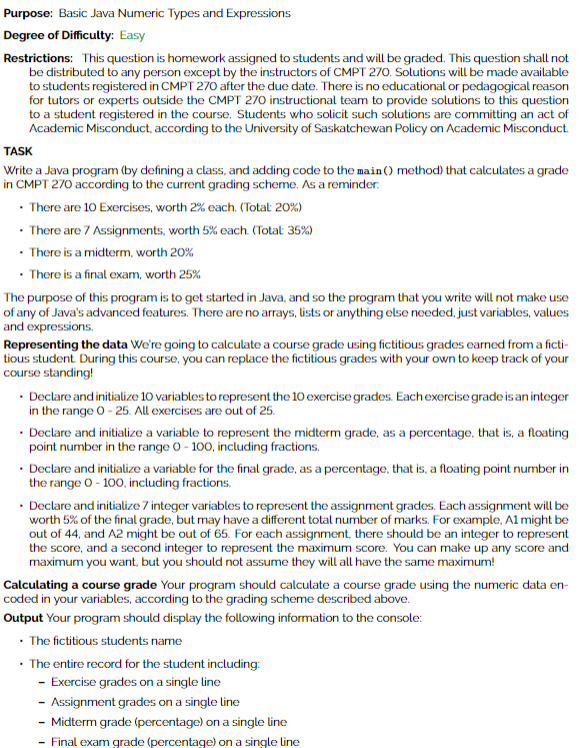
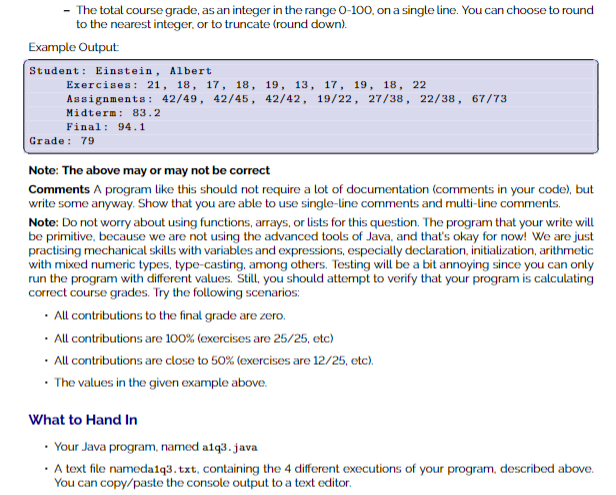
Purpose: Basic Java Numeric Types and Expressions Degree of Difficulty: Easy Restrictions: This question is homework assigned to students and will be graded. This question shall not be distributed to any person except by the instructors of CMPT 270. Solutions will be made available to students registered in CMPT 270 after the due date. There is no educational or pedagogical reason for tutors or experts outside the CMPT 270 instructional team to provide solutions to this question to a student registered in the course. Students who solicit such solutions are committing an act of Academic Misconduct, according to the University of Saskatchewan Policy on Academic Misconduct. TASK Write a Java program (by defining a class, and adding code to the main() method) that calculates a grade in CMPT 270 according to the current grading scheme. As a reminder. - There are 10 Exercises, worth 2\% each. (Total: 20\%) - There are 7 Assignments, worth 5\% each. (Total 35\%) - There is a midterm, worth \( 20 \% \) - There is a final exam, worth 25\% The purpose of this program is to get started in Java, and so the program that you write will not make use of any of Java's advanced features. There are no arrays, lists or anything else needed, just variables, values and expressions. Representing the data We're going to calculate a course grade using fictitious grades earned from a fictitious student During this course, you can replace the fictitious grades with your own to keep track of your course standing! - Declare and initialize 10 variables to represent the 10 exercise grades. Each exercise grade is an integer in the range \( \mathrm{O}-25 \). All exercises are out of \( 25 . \) - Declare and initialize a variable to represent the midterm grade, as a percentage, that is, a floating point number in the range \( \mathrm{O}-100 \), including fractions. - Declare and initialize a variable for the final grade, as a percentage, that is, a floating point number in the range \( \mathrm{O}-100 \), including fractions. - Declare and initialize 7 integer variables to represent the assignment grades. Each assignment will be worth 5\% of the final grade, but may have a different total number of marks. For example, A1 might be out of 44, and \( A 2 \) might be out of 65 . For each assignment, there should be an integer to represent the score, and a second integer to represent the maximum score. You can make up any score and maximum you want, but you should not assume they will all have the same maximum! Calculating a course grade Your program should calculate a course grade using the numeric data encoded in your variables, according to the grading scheme described above. Output Your program should display the following information to the console: - The fictitious students name - The entire record for the student including: - Exercise grades on a single line - Assignment grades on a single line - Midterm grade (percentage) on a single line - Final exam grade (percentage) on a single line
- The total course grade, as an integer in the range 0-100, on a single line. You can choose to round to the nearest integer, or to truncate (round down). Example Output Student: Einstein, A1bert Exercises: \( 21,18,17,18,19,13,17,19,18,22 \) Assignments: \( 42 / 49,42 / 45,42 / 42,19 / 22,27 / 38,22 / 38,67 / 73 \) Midterm: \( 83.2 \) Final: \( 94.1 \) Grade: 79 Note: The above may or may not be correct Comments A program like this should not require a lot of documentation (comments in your code), but write some anyway. Show that you are able to use single-line comments and multi-line comments. Note: Do not worry about using functions, arrays, or lists for this question. The program that your write will be primitive, because we are not using the advanced tools of Java, and that's okay for now! We are just practising mechanical skills with variables and expressions, especially declaration, initialization, arithmetic with mixed numeric types, type-casting. among others. Testing will be a bit annoying since you can only run the program with different values. Still, you should attempt to verify that your program is calculating correct course grades. Try the following scenarios: - All contributions to the final grade are zero. - All contributions are 100\% (exercises are 25/25, etc) - All contributions are close to \( 50 \% \) (exercises are 12/25, etc). - The values in the given example above. What to Hand In - Your Java program, named a1q3. java - A text file nameda1q3.txt, containing the 4 different executions of your program, described above. You can copy/paste the console output to a text editor.