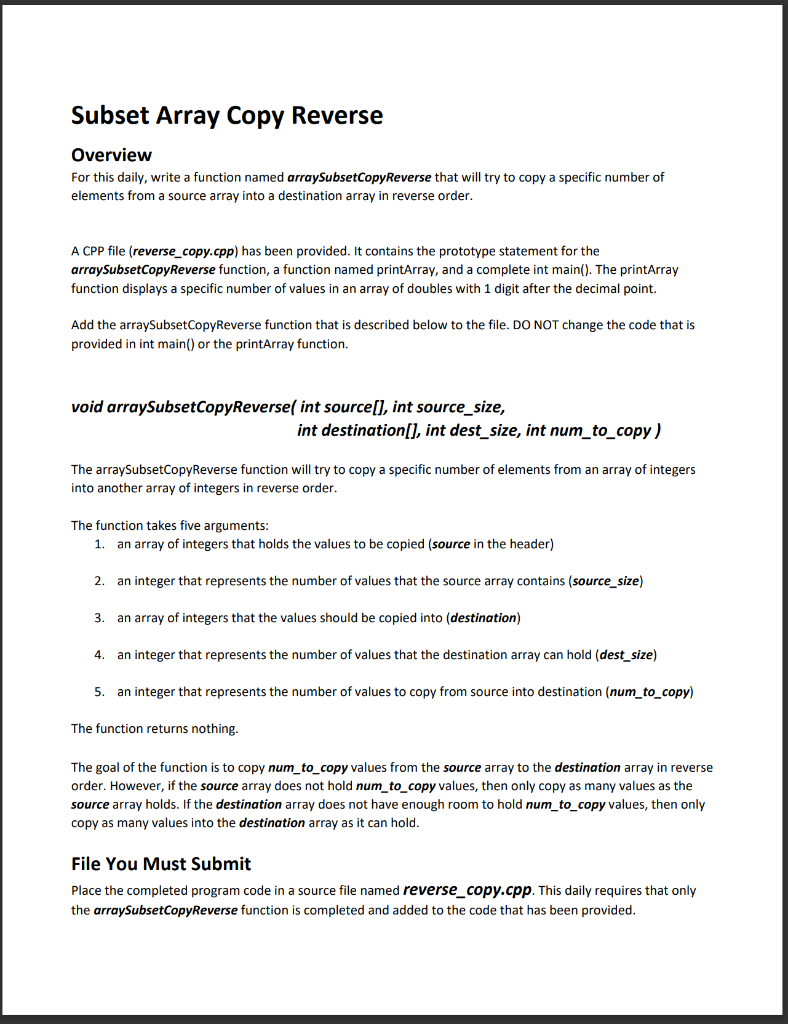
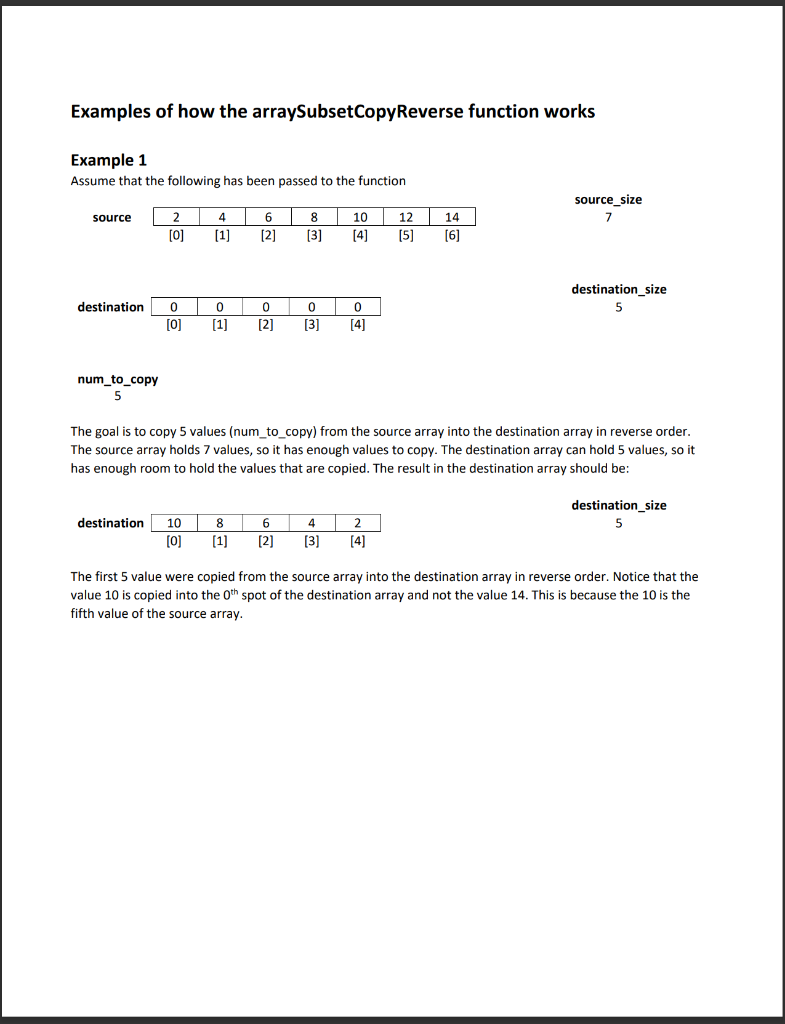
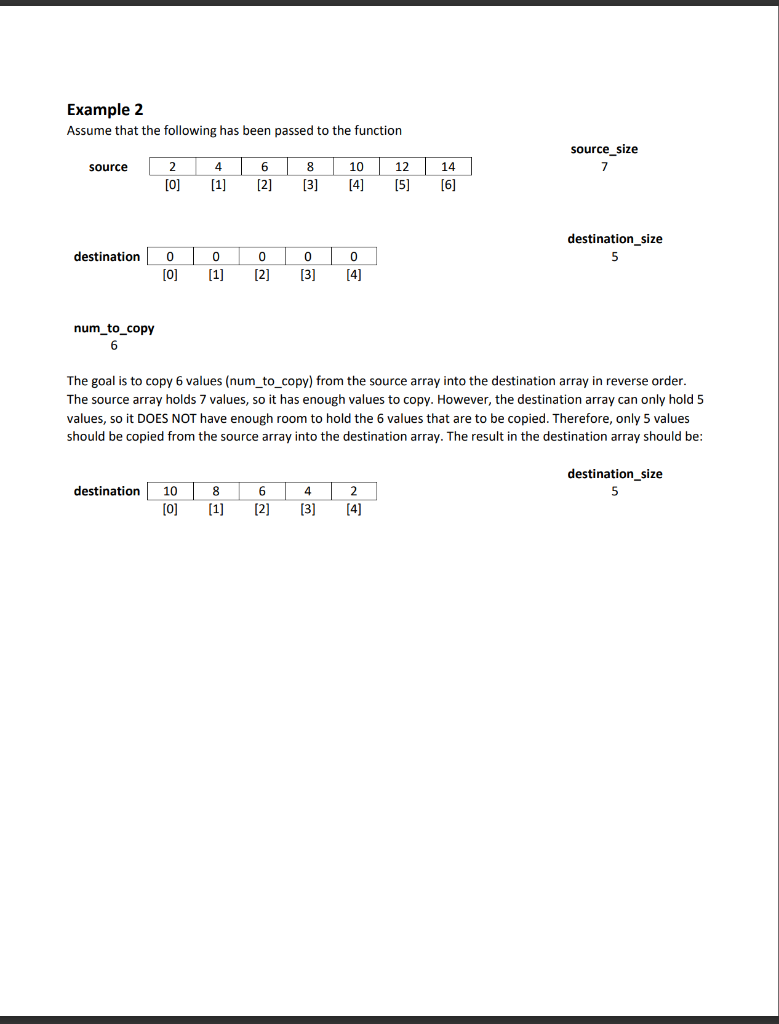
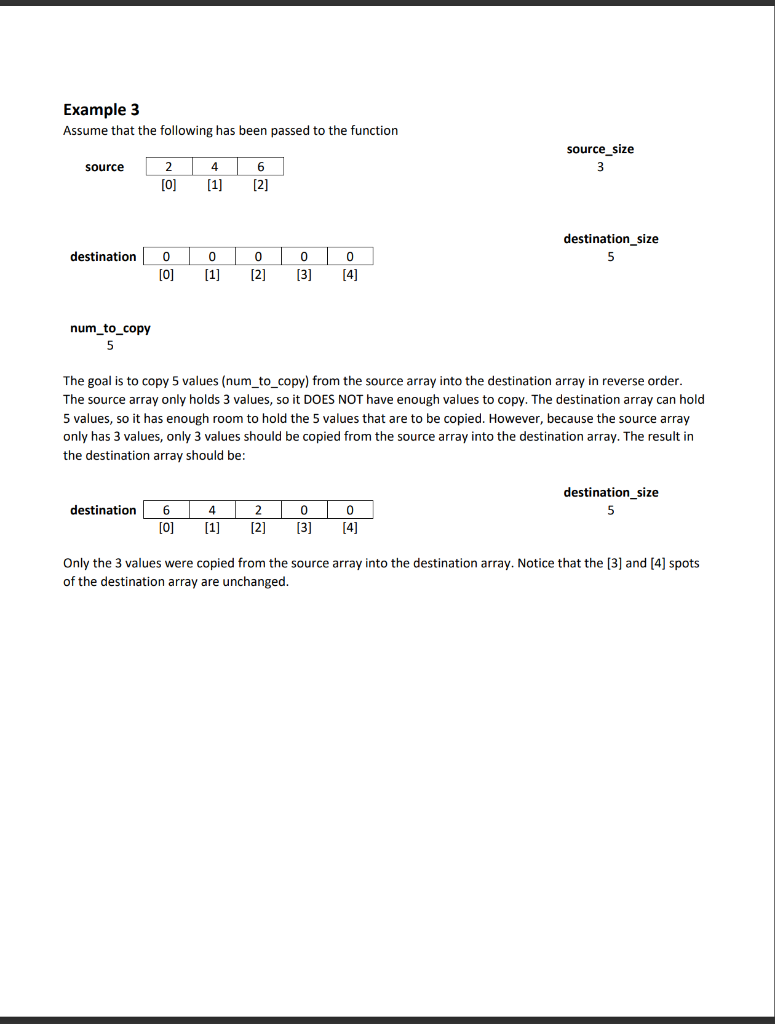
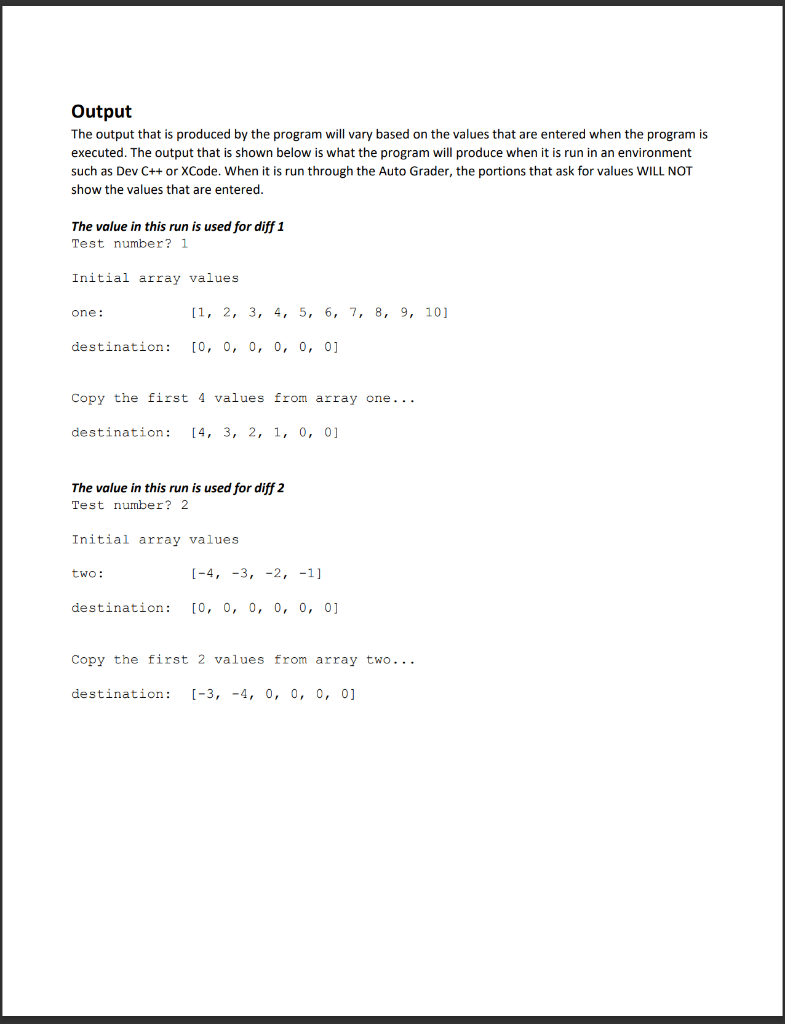
![The value in this run is used for diff 3
Test number? 3
Initial array values
one: \( [1,2,3,4,5,6,7,8,9,10] \)
destination: \](https://media.cheggcdn.com/media/6da/6dae55eb-2e49-493c-829a-4a1fafd4eeaa/php30IqK3)
#include <iostream>
#include <iomanip>
using namespace std;
#define ONE_SIZE 10
#define TWO_SIZE 4
#define DEST_SIZE 6
#define MAX_SIZE 20
void arraySubsetCopyReverse(int[], int, int[], int, int);
void printArray(int[], int);
int main()
{
int one[ONE_SIZE] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int two[TWO_SIZE] = {-4, -3, -2, -1};
int destination[DEST_SIZE] = {0};
int test_num;
cout << "Test number? ";
cin >> test_num;
//Execute tests based on the test number that was entered by the user
if(test_num == 1)
{
//Display the original values in the one and destination arrays
cout << endl << "Initial array values" << endl << endl << "one: ";
printArray(one, ONE_SIZE);
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
cout << endl << endl << "Copy the first 4 values from array one..." << endl;
//Copy 4 values from array one into the destination array
arraySubsetCopyReverse(one, ONE_SIZE, destination, DEST_SIZE, 4);
//display the updated destination array
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
}
else if(test_num == 2)
{
//Display the original values in the two and destination arrays
cout << endl << "Initial array values" << endl << endl << "two: ";
printArray(two, TWO_SIZE);
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
cout << endl << endl << "Copy the first 2 values from array two..." << endl;
//Copy 2 values from array two into the destination array
arraySubsetCopyReverse(two, TWO_SIZE, destination, DEST_SIZE, 2);
//display the updated destination array
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
}
else if(test_num == 3)
{
//Display the original values in the one and destination arrays
cout << endl << "Initial array values" << endl << endl << "one: ";
printArray(one, ONE_SIZE);
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
cout << endl << endl << "Copy all the values from array one..." << endl;
//Copy all values from array one into the destination array
arraySubsetCopyReverse(one, ONE_SIZE, destination, DEST_SIZE, ONE_SIZE);
//display the updated destination array
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
}
else if(test_num == 4)
{
//Display the original values in the two and destination arrays
cout << endl << "Initial array values" << endl << endl << "two: ";
printArray(two, TWO_SIZE);
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
cout << endl << endl << "Copy more values from array two than are defined..." << endl;
//Copy 6 values from array two into the destination array
arraySubsetCopyReverse(two, TWO_SIZE, destination, DEST_SIZE, 6);
//display the updated destination array
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
}
else if(test_num == 5)
{
//Display the original values in the one, two and destination arrays
cout << endl << "Initial array values" << endl << endl << "one: ";
printArray(one, ONE_SIZE);
cout << endl << "two: ";
printArray(two, TWO_SIZE);
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
cout << endl << endl << "Copy 6 values from array one and then 3 values from array two..." << endl;
//Copy 6 values from array one into the destination array
arraySubsetCopyReverse(one, ONE_SIZE, destination, DEST_SIZE, 6);
arraySubsetCopyReverse(two, TWO_SIZE, destination, DEST_SIZE, 3);
//display the updated destination array
cout << endl << "destination: ";
printArray(destination, DEST_SIZE);
}
else
{
int bigger_dest[MAX_SIZE] = {0};
int three[MAX_SIZE] = {2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 1, 3, 5, 7, 9, 11, 13, 15, 17, 19};
//Display the original values in the one, two and destination arrays
cout << endl << "Initial array values" << endl << endl << "two: ";
printArray(two, TWO_SIZE);
cout << endl << "three: ";
printArray(three, MAX_SIZE);
cout << endl << "bigger_dest: ";
printArray(bigger_dest, MAX_SIZE);
cout << endl << endl << "Copy 15 values from array three..." << endl;
//Copy 15 values from array three into the bigger_dest array
arraySubsetCopyReverse(three, MAX_SIZE, bigger_dest, MAX_SIZE, 15);
//display the updated destination array
cout << endl << "bigger_dest: ";
printArray(bigger_dest, MAX_SIZE);
cout << endl << endl << "Copy 15 values from array two..." << endl;
//Copy 15 values from array two into the bigger_dest array
arraySubsetCopyReverse(two, TWO_SIZE, bigger_dest, MAX_SIZE, 15);
//display the updated destination array
cout << endl << "bigger_dest: ";
printArray(bigger_dest, MAX_SIZE);
}
return 0;
}
//This function display an array of integers
void printArray(int a[], int l)
{
int i;
//Display a leading [ and all but the last value
cout << "[";
for (i = 0; i < l-1; i ++)
cout << a[i] << ", ";
//Display the last value in the array and the ending ]
cout << a[l-1] << "]" << endl;
} // printArray()
Subset Array Copy Reverse Overview For this daily, write a function named arraySubsetCopyReverse that will try to copy a specific number of elements from a source array into a destination array in reverse order. A CPP file (reverse_copy.cpp) has been provided. It contains the prototype statement for the arraySubsetCopyReverse function, a function named printArray, and a complete int main(). The printArray function displays a specific number of values in an array of doubles with 1 digit after the decimal point. Add the arraySubsetCopyReverse function that is described below to the file. DO NOT change the code that is provided in int main() or the printArray function. void arraySubsetCopyReverse( int source[], int source_size, int destination[], int dest_size, int num_to_copy ) The arraySubsetCopyReverse function will try to copy a specific number of elements from an array of integers into another array of integers in reverse order. The function takes five arguments: 1. an array of integers that holds the values to be copied (source in the header) 2. an integer that represents the number of values that the source array contains (source_size) 3. an array of integers that the values should be copied into (destination) 4. an integer that represents the number of values that the destination array can hold (dest_size) 5. an integer that represents the number of values to copy from source into destination (num_to_copy) The function returns nothing. The goal of the function is to copy num_to_copy values from the source array to the destination array in reverse order. However, if the source array does not hold num_to_copy values, then only copy as many values as the source array holds. If the destination array does not have enough room to hold num_to_copy values, then only copy as many values into the destination array as it can hold. File You Must Submit Place the completed program code in a source file named reverse_copy.cpp. This daily requires that only the arraySubsetCopyReverse function is completed and added to the code that has been provided.
Examples of how the arraySubsetCopyReverse function works Example 1 Assume that the following has been passed to the function \( \begin{array}{cc}\text { source } & \begin{array}{c}\text { source_size } \\ 7\end{array} \\ \text { destination } & \text { destination_size } \\ 5\end{array} \) \[ \text { num_to_copy } \] The goal is to copy 5 values (num_to_copy) from the source array into the destination array in reverse order. The source array holds 7 values, so it has enough values to copy. The destination array can hold 5 values, so it has enough room to hold the values that are copied. The result in the destination array should be: destination_size destination The first 5 value were copied from the source array into the destination array in reverse order. Notice that the value 10 is copied into the \( 0^{\text {th }} \) spot of the destination array and not the value 14 . This is because the 10 is the fifth value of the source array.
Example 2 Assume that the following has been passed to the function \( \begin{array}{cc}\text { source } & \text { source_size } \\ 7 \\ \text { destinatiot } & \text { destination_size } \\ 5\end{array} \) \[ \text { num_to_copy } \] The goal is to copy 6 values (num_to_copy) from the source array into the destination array in reverse order. The source array holds 7 values, so it has enough values to copy. However, the destination array can only hold 5 values, so it DOES NOT have enough room to hold the 6 values that are to be copied. Therefore, only 5 values should be copied from the source array into the destination array. The result in the destination array should be:
Example 3 Assume that the following has been passed to the function source source_size 3 destination_size destinatic \[ \text { num_to_copy } \] The goal is to copy 5 values (num_to_copy) from the source array into the destination array in reverse order. The source array only holds 3 values, so it DOES NOT have enough values to copy. The destination array can hold 5 values, so it has enough room to hold the 5 values that are to be copied. However, because the source array only has 3 values, only 3 values should be copied from the source array into the destination array. The result in the destination array should be: destination_size destination 5 Only the 3 values were copied from the source array into the destination array. Notice that the [3] and [4] spots of the destination array are unchanged.
Output The output that is produced by the program will vary based on the values that are entered when the program is executed. The output that is shown below is what the program will produce when it is run in an environment such as Dev C++ or XCode. When it is run through the Auto Grader, the portions that ask for values WILL NOT show the values that are entered. The value in this run is used for diff 1 Test number? 1 Initial array values one: \( [1,2,3,4,5,6,7,8,9,10] \) destination: \( [0,0,0,0,0,0] \) Copy the first 4 values from array one... destination: \( [4,3,2,1,0,0] \) The value in this run is used for diff 2 Test number? 2 Initial array values two: \( [-4,-3,-2,-1] \) destination: \( [0,0,0,0,0,0] \) Copy the first 2 values from array two... destination: \( [-3,-4,0,0,0,0] \)
The value in this run is used for diff 3 Test number? 3 Initial array values one: \( [1,2,3,4,5,6,7,8,9,10] \) destination: \( [0,0,0,0,0,0] \) Copy all the values from array one... destination: \( [6,5,4,3,2,1] \) The value in this run is used for diff 4 Test number? 4 Initial array values two: \( [-4,-3,-2,-1] \) destination: \( [0,0,0,0,0,0] \) Copy more values from array two than are defined... destination: \( [-1,-2,-3,-4,0,0] \)