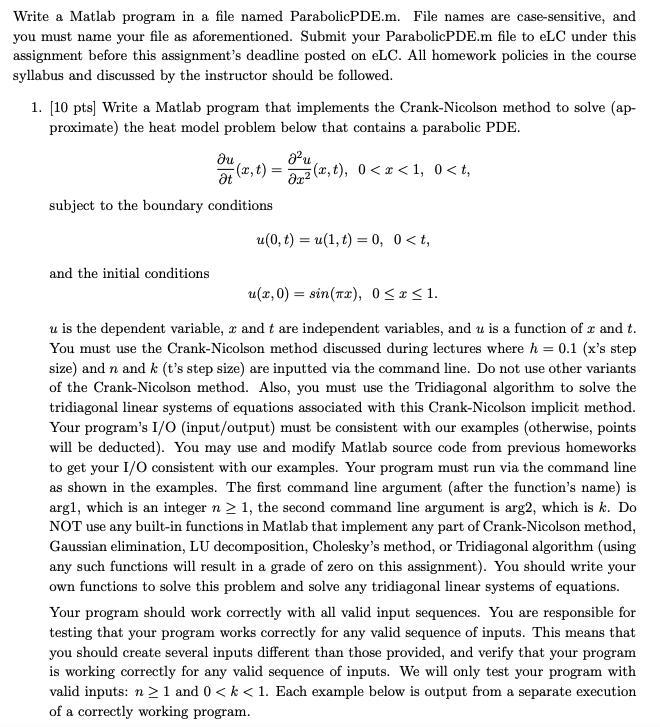
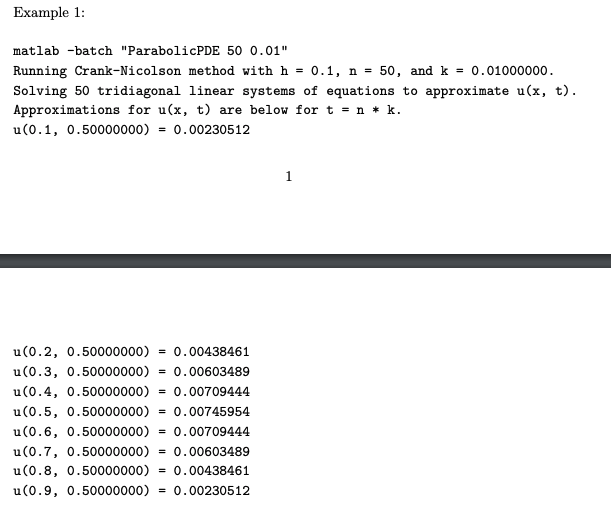
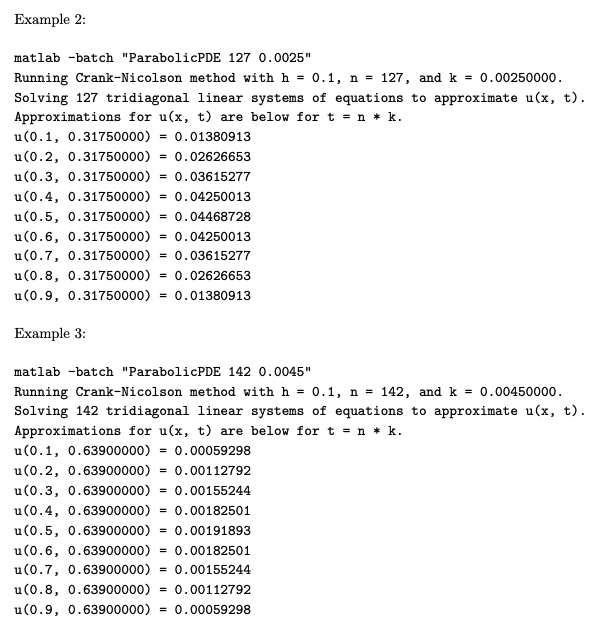
I would like a MATLAB code that prints as shown in examples! Thank you!
Write a Matlab program in a file named ParabolicPDE.m. File names are case-sensitive, and you must name your file as aforementioned. Submit your ParabolicPDE.m file to eLC under this assignment before this assignment's deadline posted on eLC. All homework policies in the course syllabus and discussed by the instructor should be followed. 1. [10 pts] Write a Matlab program that implements the Crank-Nicolson method to solve (approximate) the heat model problem below that contains a parabolic PDE. ?t?u?(x,t)=?x2?2u?(x,t),0<x<1,0<t, subject to the boundary conditions u(0,t)=u(1,t)=0,0<t and the initial conditions u(x,0)=sin(?x),0?x?1 u is the dependent variable, x and t are independent variables, and u is a function of x and t. You must use the Crank-Nicolson method discussed during lectures where h=0.1 (x's step size) and n and k (t's step size) are inputted via the command line. Do not use other variants of the Crank-Nicolson method. Also, you must use the Tridiagonal algorithm to solve the tridiagonal linear systems of equations associated with this Crank-Nicolson implicit method. Your program's I/O (input/output) must be consistent with our examples (otherwise, points will be deducted). You may use and modify Matlab source code from previous homeworks to get your I/O consistent with our examples. Your program must run via the command line as shown in the examples. The first command line argument (after the function's name) is arg1, which is an integer n?1, the second command line argument is arg2, which is k. Do NOT use any built-in functions in Matlab that implement any part of Crank-Nicolson method, Gaussian elimination, LU decomposition, Cholesky's method, or Tridiagonal algorithm (using any such functions will result in a grade of zero on this assignment). You should write your own functions to solve this problem and solve any tridiagonal linear systems of equations. Your program should work correctly with all valid input sequences. You are responsible for testing that your program works correctly for any valid sequence of inputs. This means that you should create several inputs different than those provided, and verify that your program is working correctly for any valid sequence of inputs. We will only test your program with valid inputs: n?1 and 0<k<1. Each example below is output from a separate execution of a correctly working program.
Example 1: matlab -batch "ParabolicPDE 500.01 " Running Crank-Nicolson method with h=0.1,n=50, and k=0.01000000. Solving 50 tridiagonal linear systems of equations to approximate u(x,t). Approximations for u(x,t) are below for t=n?k. u(0.1,0.50000000)=0.00230512 1 u(0.2,0.50000000)=0.00438461 u(0.3,0.50000000)=0.00603489 u(0.4,0.50000000)=0.00709444 u(0.5,0.50000000)=0.00745954 u(0.6,0.50000000)=0.00709444 u(0.7,0.50000000)=0.00603489 u(0.8,0.50000000)=0.00438461 u(0.9,0.50000000)=0.00230512
Example 2: matlab -batch "ParabolicPDE 127 0.0025" Running Crank-Nicolson method with h=0.1,n=127, and k=0.00250000. Solving 127 tridiagonal linear systems of equations to approximate u(x,t). Approximations for u(x,t) are below for t=n?k. u(0.1,0.31750000)=0.01380913 u(0.2,0.31750000)=0.02626653 u(0.3,0.31750000)=0.03615277 u(0.4,0.31750000)=0.04250013 u(0.5,0.31750000)=0.04468728 u(0.6,0.31750000)=0.04250013 u(0.7,0.31750000)=0.03615277 u(0.8,0.31750000)=0.02626653 u(0.9,0.31750000)=0.01380913 Example 3: matlab -batch "ParabolicPDE 142 0.0045" Running Crank-Nicolson method with h=0.1,n=142, and k=0.00450000. Solving 142 tridiagonal linear systems of equations to approximate u(x,t). Approximations for u(x,t) are below for t=n?k. u(0.1,0.63900000)=0.00059298 u(0.2,0.63900000)=0.00112792 u(0.3,0.63900000)=0.00155244 u(0.4,0.63900000)=0.00182501 u(0.5,0.63900000)=0.00191893 u(0.6,0.63900000)=0.00182501 u(0.7,0.63900000)=0.00155244 u(0.8,0.63900000)=0.00112792 u(0.9,0.63900000)=0.00059298