CODE IN PYTHON PLS!???????
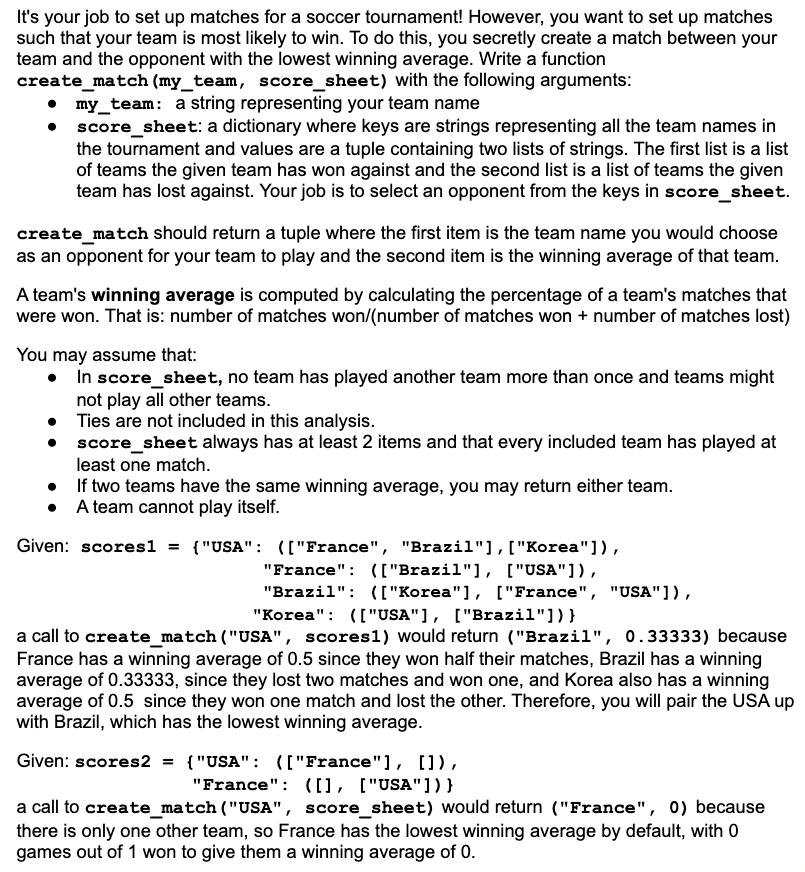
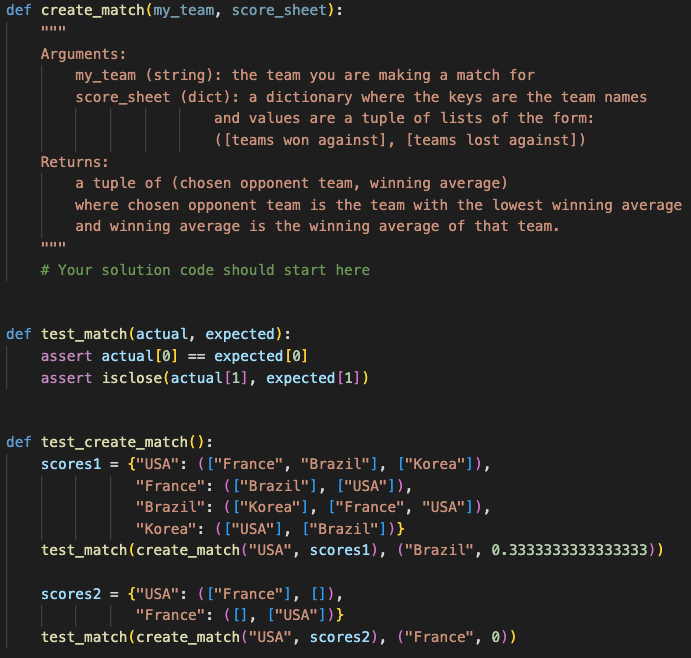
Requirements:
???????
It's your job to set up matches for a soccer tournament! However, you want to set up matches such that your team is most likely to win. To do this, you secretly create a match between your team and the opponent with the lowest winning average. Write a function create_match (my_team, score_sheet) with the following arguments: - my_team: a string representing your team name - score_sheet: a dictionary where keys are strings representing all the team names in the tournament and values are a tuple containing two lists of strings. The first list is a list of teams the given team has won against and the second list is a list of teams the given team has lost against. Your job is to select an opponent from the keys in score_sheet. create_match should return a tuple where the first item is the team name you would choose as an opponent for your team to play and the second item is the winning average of that team. A team's winning average is computed by calculating the percentage of a team's matches that were won. That is: number of matches won/(number of matches won + number of matches lost) You may assume that: - In score_sheet, no team has played another team more than once and teams might not play all other teams. - Ties are not included in this analysis. - score_sheet always has at least 2 items and that every included team has played at least one match. - If two teams have the same winning average, you may return either team. - A team cannot play itself. Given: scores1 \( =\{ \) "USA": (["France", "Brazil"], ["Korea"]), "France": (["Brazil"], ["USA"]), "Brazil": (["Korea"], ["France", "USA"]), "Korea": (["USA"], ["Brazil"])\} a call to create_match("USA", scores1) would return ("Brazil", \( 0.33333 \) ) because France has a winning average of \( 0.5 \) since they won half their matches, Brazil has a winning average of \( 0.33333 \), since they lost two matches and won one, and Korea also has a winning average of \( 0.5 \) since they won one match and lost the other. Therefore, you will pair the USA up with Brazil, which has the lowest winning average. Given: scores2 = \{"USA": (["France"], []), "France": ([], ["USA"])\} a call to create_match ("USA", score_sheet) would return ("France", 0 ) because there is only one other team, so France has the lowest winning average by default, with 0 games out of 1 won to give them a winning average of 0 . You may only use parts of Python that have been covered in the class (defined as found on lecture slides or section handouts or discussed in lecture). - In particular, you MAY use these items discussed during the last weeks of the quarter: list comprehensions, get and setdefault, enumerate, and ternary assignment. You do not NEED to use any of these to solve these problems, and we will not be awarding any extra points for using them, but you are welcome to use them. - You also MAY use the functions min, max, and sum. - You MAY write and call helper functions(but this is not expected/required) - You do NOT need classes for any problems in Part 1. - You MAY import itemgetter and use it. - You may NOT use anything from the Math module other than isclose, which has lareayd been imported. - You may NOT use things we have not covered, such as recursion or lambda functions.