Can someone help with this program in python
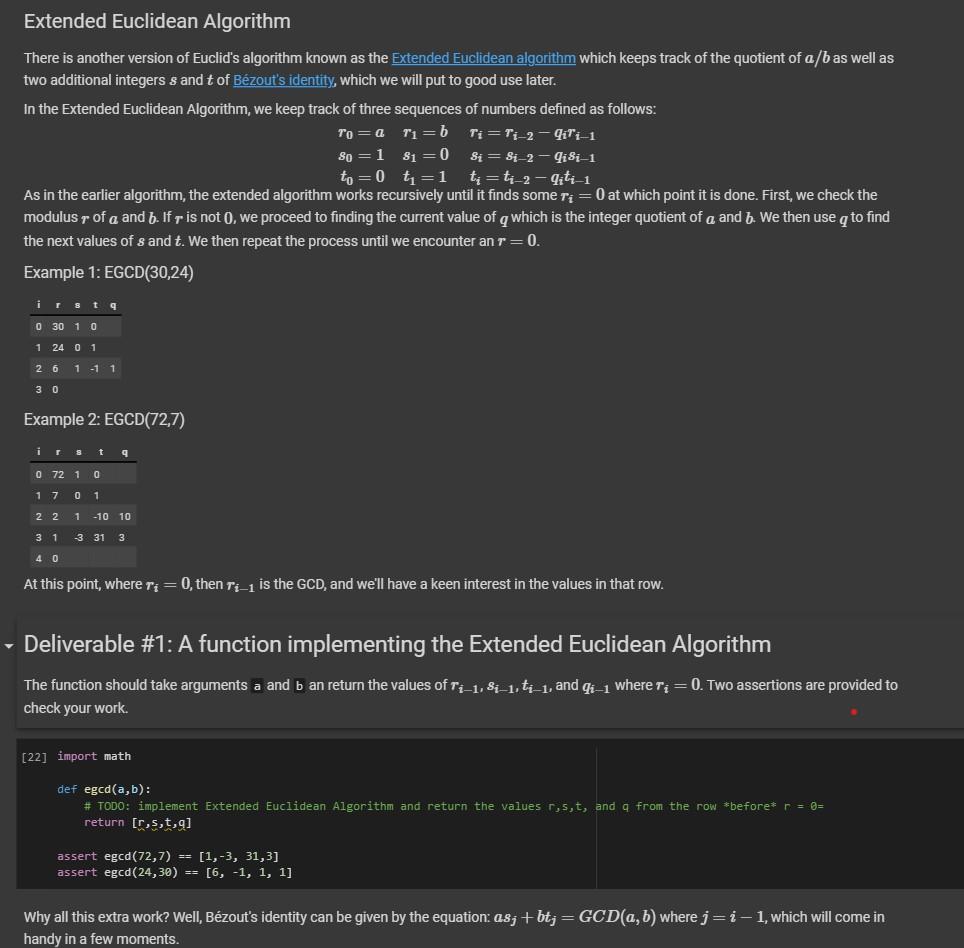
There is another version of Euclid's algorithm known as the Extended Euclidean algorithm which keeps track of the quotient of a/b as well as two additional integers s and t of Bézout's identity, which we will put to good use later. In the Extended Euclidean Algorithm, we keep track of three sequences of numbers defined as follows: r0?=as0?=1t0?=0?r1?=bs1?=0t1?=1?ri?=ri?2??qi?ri?1?si?=si?2??qi?si?1?ti?=ti?2??qi?ti?1?? As in the earlier algorithm, the extended algorithm works recursively until it finds some ri?=0 at which point it is done. First, we check the modulus r of a and b. If r is not 0, we proceed to finding the current value of q which is the integer quotient of a and b. We then use q to find the next values of s and t. We then repeat the process until we encounter an r=0. Example 1: EGCD(30,24) Example 2: EGCD(72,7) At this point, where ri?=0, then ri?1? is the GCD, and we'll have a keen interest in the values in that row. Deliverable \#1: A function implementing the Extended Euclidean Algorithm The function should take arguments a and b an return the values of ri?1?,si?1?,ti?1?, and qi?1? where ri?=0. Two assertions are provided to check your work. [22] import math def egcd (a,b) : \# TODO: implement Extended Euclidean Algorithm and return the values r, 5,t, and q from the row ? before* r=0= return [r,5,t,q] assert egcd(72,7)=[1,?3,31,3] assert egcd(24,30)=[6,?1,1,1] Why all this extra work? Well, Bézout's identity can be given by the equation: asj?+btj?=GCD(a,b) where j=i?1, which will come in handy in a few moments.