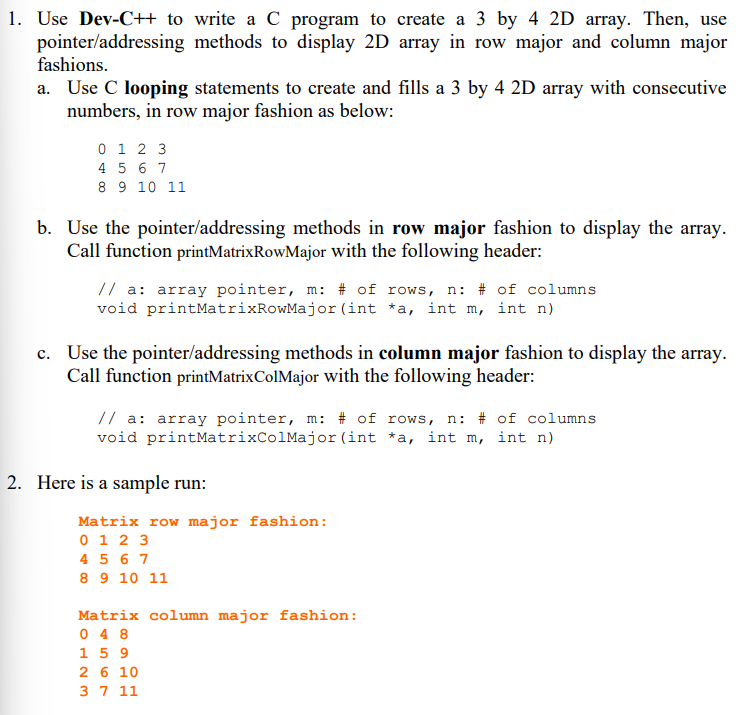
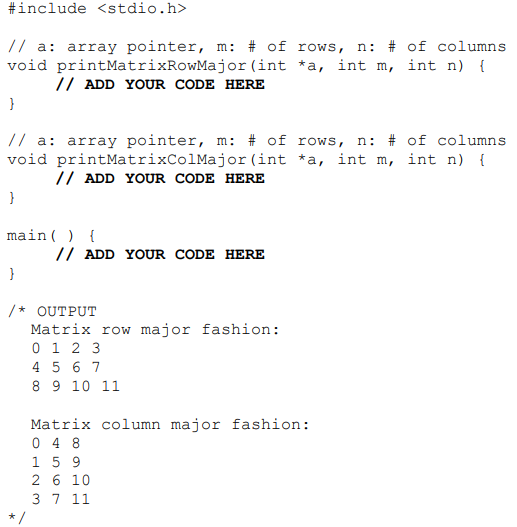
1. Use Dev-C++ to write a C program to create a 3 by 4 2D array. Then, use methods to display 2D array in row major and column major pointer/addressing fashions. a. Use C looping statements to create and fills a 3 by 4 2D array with consecutive numbers, in row major fashion as below: 0 1 2 3 4 5 6 7 8 9 10 11 b. Use the pointer/addressing methods in row major fashion to display the array. Call function printMatrixRowMajor with the following header: //a: array pointer, m: # of rows, n: # of columns void printMatrixRowMajor (int *a, int m, int n) c. Use the pointer/addressing methods in column major fashion to display the array. Call function printMatrixColMajor with the following header: // a: array pointer, m: # of rows, n: # of columns void printMatrixColMajor (int *a, int m, int n) Matrix row major fashion: 0 1 2 3 4 5 6 7 8 9 10 11 Matrix column major fashion: 048 159 2 6 10 3 7 11 2. Here is a sample run:
#include // a: array pointer, m: # of rows, n: # of columns void printMatrixRowMajor (int *a, int m, int n) { // ADD YOUR CODE HERE } // a: array pointer, m: # of rows, n: # of columns void printMatrixColMajor (int *a, int m, int n) { // ADD YOUR CODE HERE } main() { // ADD YOUR CODE HERE } /* OUTPUT Matrix row major fashion: 0 1 2 3 4567 8 9 10 11 Matrix column major fashion: 048 159 2 6 10 3 7 11 */